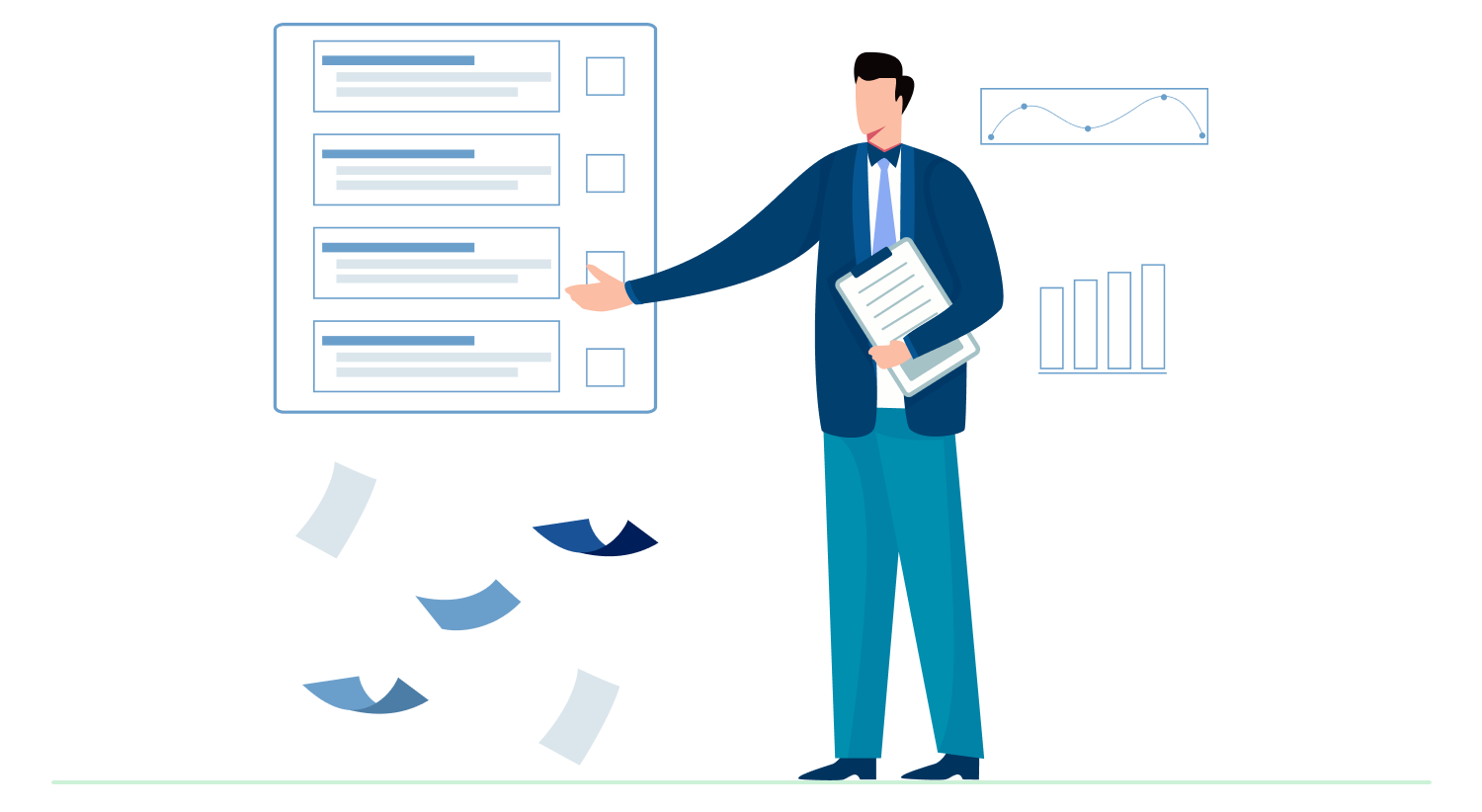
What Is Refactoring?
Refactoring Definition
Refactoring involves modifying an application’s source code without altering its external behavior. Refactoring aims to enhance certain nonfunctional aspects of the code, such as readability, complexity, maintainability, and extensibility.
Have you ever cleaned your room and reorganized things not because you have broken anything but just to make it neater and easier to find what you need? Code refactoring is similar – it’s a way to clean up and restructure your code to improve its maintainability and quality without changing its external functionality.
Refactoring means changing the internal structure of code to make it easier to work with down the road. Renaming variables to be more descriptive, consolidating duplicate code, or reorganizing files can go a long way in keeping code tidy and readable. By taking the time to refactor periodically, developers can prevent technical debt from accumulating and make future enhancements or bug fixes much simpler.
Some key benefits of refactoring include:
- Improved code quality: Removing duplication, unnecessary complexity, and poorly written code results in a cleaner and more coherent structure.
- Increased maintainability: Well-organized, intention-revealing code is easier to understand and modify in the future as needs change.
- Better performance: Reducing redundant operations and unnecessary files/code makes the application more efficient.
- Lower risk of bugs: Refactored code containing fewer flaws and inconsistencies is less prone to bugs introduced during development.
- Higher developer productivity: Time spent understanding code gets minimized, allowing developers to focus on new features instead of deciphering messy code.
Some common refactoring techniques include extracting methods to condense logic, renaming variables for clarity, consolidating duplicate code, restructuring file hierarchies, and simplifying conditional expressions. Larger refactors may involve techniques like abstraction to remove repetition at a higher level.
Refactorings are typically small, incremental changes tested after each one to avoid unintended consequences. With regular refactoring habits, developers can prevent their code from becoming disorganized over time and more enjoyable to extend in the future. Clean code leads to better software in the long run.
Why Refactor?
Code refactoring is essential to keep code clean, readable, and maintainable over time. As developers work on a codebase, natural changes can introduce inefficiencies, redundancies, and complexity if not appropriately addressed.
Some common reasons code becomes messy and requires refactoring include:
- Redundant code creeps in as developers duplicate logic to solve problems quickly. This wastes time and effort in maintaining duplicate segments that should get consolidated.
- Variables and methods accumulate that no longer serve a clear purpose. Outdated code obscures the intended functionality.
- Functions and classes expand indefinitely as new requirements get added without undergoing redesign. This results in sprawling code that is challenging to navigate and comprehend.
- Quick fixes get applied through unnecessary conditions and loops instead of taking the time for a cleaner design. This practice litters the code with clutter.
- Over time, the initial design shows limitations, but lack of refactoring prevents improvement. Technical debt rises, and technical solutions become messy.
Failing to refactor carries real negative consequences for code quality and project health over the long run:
- Technical debt snowballs as each small unrefactored change compounds complexity, ultimately strangling a codebase and hindering productivity.
- Missed deadlines can occur if developers spend too much time unraveling debt that should have received earlier attention through lightweight, incremental refactoring.
- Rashly restructuring large chunks of code brings risks if not guided by adequate tests, potentially introducing hard-to-find bugs.
- Quality erodes as unattended issues accumulate, making code difficult to work with, understand, and scale. It threatens the ultimate success and stability of an application.
In summary, periodic refactoring is necessary to manage complexity, eliminate defects, improve design, and keep code healthy for ongoing collaboration and long-term maintenance. A proactive approach to refactoring pays dividends throughout a project’s lifespan.
When Is the Best Time to Refactor Code?
Here are some elaborated guidelines for when the best times are to refactor code:
Continuous but Purposeful Refactoring
Implementing refactoring in small, incremental changes allows maintaining clean code over the long run.
After each minor feature addition or bug fix, developers should assess ways to simplify the code through extraction, renaming, or restructuring. However, refactoring for its own sake provides little value and distracts from pressing work. To enable future growth, the goal is to improve design qualities like readability, flexibility, and testability.
Before Major Updates or Features
Tackling larger refactors in advance of significant modifications establishes a sturdy base.
For example, consolidating duplicated logic or extracting complex conditional checks readies the code for introductions like new endpoints, data models, or payment integrations. Refactored code more seamlessly absorbs changes without introducing hard-to-track bugs or regressions.
Familiarizing with Inherited Code
Stepping into an unfamiliar codebase requires understanding how it works. Refactoring assists comprehension by eliminating redundancies, clarifying purposes, and standardizing styles. It eases maintenance and collaboration for all developers going forward. Additionally, it identifies low-hanging opportunities to enhance the architecture.
Following Bug or Performance Issues
An influx of similar defects implies code quality debts. Refactoring to resolve underlying design flaws prevents recurrence.
For example, high memory usage may stem from inappropriate data structures. Generalizing common condition patterns may curb edge case errors. Metrics guide focusing on refactoring where it prevents future pains.
During Code Reviews
Peers routinely examine code for quality, best practices, and readability. Refactoring proposals improves shared code ownership. For instance, a reviewer may note redundant validation logic across files that could get consolidated. Addressing such opportunities when fresh prevents technical debts from ballooning.
Refactoring requires balance – tackling specific pain points while avoiding distractions from primary work. The best times maximize value through a foundation for future changes.
6 Common Refactoring Techniques
Here are the most common refactoring techniques you need to know about:
1. Red-Green-Refactor
The Red-Green-Refactor technique is one of the most fundamental and practical refactoring practices used in Test-Driven Development (TDD). It promotes a development workflow focusing on quality, sustainability, and reducing risks associated with code changes.
The process begins with the “Red” step, where a developer writes an automated test that fails or is “Red.” It validates that the test is appropriately identifying a missing feature or bug. No code has been written at this stage apart from the test itself.
Once the test fails, the developer then moves to the “Green” phase. At this point, the minimum code is written only to satisfy the test and turn its status from failing to passing or “Green.” Readability and long-term design are not a priority here – the sole goal is to pass the test as simply as possible.
After the test turns Green, the refactoring or “Refactor” stage begins. With the safety net of the now passing test, the developer can freely change and improve the code without fear of breaking functionality. You can address things like removing duplication, simplifying conditional logic, and improving names and documentation.
2. Refactoring by Abstraction
Refactoring by abstraction focuses on leveraging object-oriented principles like inheritance, polymorphism, and composition to reduce duplication and improve structure in codebases that utilize classes and hierarchies. This technique aims to achieve a cleaner separation of concerns through abstraction.
Two fundamental refactoring patterns are the “pull up method” and the “push down method.”
The pull-up method identifies standard functionality shared between subclasses and extracts it into the parent superclass. It eliminates duplicate code by abstracting the shared behavior to a single implementation in the superclass.
The push-down method is the opposite – it takes functionality defined in a superclass. It moves it down into the most specific subclasses where it applies. This places responsibility closer to where it is truly needed rather than keeping it generalized.
The class structure can get optimized by strategically applying these patterns to reflect real-world relationships and responsibilities between entities properly. It results in slimmer subclasses that focus only on their specialization rather than having mixed concerns.
3. Composing
As programs grow in size and complexity, it’s easy for your code to become disjointed and difficult to follow. Methods stretch on for dozens of lines with nested logic flows. This makes code hard to understand at a glance and problematic to maintain over time.
The composing method approach aims to tame overgrown code by breaking it into logical, cohesive chunks. You will extract discrete elements and arrange them in a clear structure. The goal is elegant simplicity through organization.
You start by identifying fragments within long methods that serve a single purpose. Anything extracting business rules, calculations, or discrete tasks is a candidate. Then, you lift these fragments into new, intention-revealing methods and replace the original code with a call to the new method.
This “extract method” refactor leads to self-documenting code by assigning semantic names to functional elements. It also cuts down on duplication by consolidating shared logic in reusable pieces.
Occasionally, you may find methods doing too little. Simply passing data between other methods. In such cases, you can perform the opposite “inline method” refactor, merging the method body into its callers for a more streamlined flow.
By applying composition strategically, your code becomes easier to navigate, understand, and evolve.
4. Simplifying Methods
Here are two techniques for simplifying methods through refactoring:
Simplifying Conditional Expressions
As programs evolve, conditional logic can become complex and convoluted over time. This makes the code difficult to follow and understand. Various refactors can help simplify conditionals.
Some examples include consolidating duplicate checks, decomposing large conditional blocks into smaller focused methods, replacing conditionals with polymorphism to distribute logic more cleanly, removing unneeded control flags, and using guard clauses to flatten nested if/else structures.
The goal is to make the intent and flow of each conditional block clear at a glance by splitting concerns, removing duplication, and distributing code responsibilities more intuitively.
Simplifying Method Calls
Method calls also benefit from simplification. Refactoring the interaction between classes can streamline interfaces and dependencies.
Parameters are an area ripe for refactoring. Removing unneeded parameters, introducing explicit methods in place of parameters, or parameterizing methods based on contexts can all cleanup signatures.
Other examples are making queries or data retrieval separate from logic methods, preserving complete objects versus splitting properties across calls, and removing setter methods if property mutation isn’t needed externally.
These refactors make method interactions leaner, clearer, and more maintainable over time. Code understanding gets promoted through simplifying at the method level.
5. Moving Features Between Objects
As software systems evolve, class boundaries can become blurred over time. Responsibilities shift, and certain classes may end up with too many concerns competing within them. It leads to code that’s difficult to comprehend and change.
Redistributing features to new homes is one way to untangle these knots and regain clarity and maintainability. The goal is to have each class solely focused on one clear role. Classes should keep closely related logic together while distributing unrelated parts to more appropriate owners.
Some signs it’s time to consider refactoring include a “God class” doing too much, methods relying heavily on external data hinting they belong elsewhere, duplicated code across classes suggesting shared abstractions, or classes tightly coupling unrelated ones.
Refactoring techniques like extracting a class, moving methods or fields, and inlining unnecessary classes help realign responsibilities judiciously. For example, pulling a discrete behavior into its class consolidates associated code and hides implementation details.
By identifying logical categories of behavior, you can thoughtfully redistribute capabilities to achieve high cohesion and loose coupling. It disentangles knots that previously made the design rigid and fragile to change.
The net result cleans up classes’ single responsibilities while simplifying dependencies and inter-class relationships. This refactored architecture proves more robust and evolvable as requirements change over time. It’s an essential technique for maintaining clean object-oriented designs.
6. Preparatory Refactoring
The underlying codebase evolves over time as software engineers continuously add new capabilities to an application. Minor design compromises can accumulate into more significant quality issues over time if not addressed.
This is where preparatory refactoring provides value. By taking the time to thoughtfully restructure code during the early stages of a new feature, developers set the project up for long-term success rather than technical debt.
Even if end users don’t directly see these behind-the-scenes refactors, they yield enormous benefits for the development team. Identifying and addressing emerging code smells beforehand makes the code flexible and extensible as requirements change.
Preparatory refactoring helps streamline the implementation of the upcoming feature by cleaning up dependencies, complexity, and duplication that could otherwise hinder progress. It promotes writing high-quality code from the beginning rather than bolting on hacks later.
With such preventative maintenance, developers avoid getting mired in infrastructure issues and can focus on core functionality. Reducing bugs and maintenance costs occurs throughout the lifetime of the project.
Best Practices on How to Refactor Code
Here are five best practices for refactoring code:
Collaboration is Key
Bringing quality assurance experts into the process ensures changes don’t undermine functionality. Testing by others catches issues developers may miss. Early QA involvement validates optimizations that don’t degrade stability.
Automate for Accuracy
Conducting manual refactoring at scale poses the risk of human error. Streamlining improvements and minimizing flaws can be achieved by integrating code analyzers, linters, and automated tests. Tools surface weak spots, enforce standards, and verify that refactors meet expectations consistently.
Take it Step-by-Step
Trying to fix everything at once invites problems. Focusing narrowly, one refinement at a time allows tracking progress and easily pinpointing defects. Iterating on small, singular tweaks maintains code health throughout versus overhaul interruptions.
Isolate Troubleshooting
Separating refactoring from debugging prevents crossover confusion. Refinements aim to clarify code’s shape, while debugging seeks root causes. Addressing known bugs first, then optimizing, prevents distraction. New breaks emerge from refactors alone for straightforward resolution.
Deduplicate Diligently
Removing redundant logic simplifies consistency and reduces work. Prioritizing deduplication achieves this key goal by extracting common patterns into shared pieces. Extracted modules foster understandability and adaptability long-term.
In moderation, discipline, and cross-involvement, refactoring uplifts quality without surprise damage when practiced with these experienced techniques.
In conclusion, just as regularly decluttering and organizing your living space makes daily life less stressful, regularly refactoring code has numerous benefits. Well-structured and easy-to-understand code is a joy to work with – you can find what you need quickly and implement new features without disruptive rewrites.
On the other hand, code left untended soon becomes cluttered with unnecessary complexity, duplication, and outdated practices. This leads developers to feel frustrated navigating an increasingly chaotic codebase.
Refactoring prevents these issues by removing clutter, improving structure, and bringing code up to current standards in a controlled way. Taking the time for regular refactoring sessions keeps a project’s codebase tidy, sustainable, and stress-free to evolve.
FAQs
ChatGPT can provide guidance and suggestions on code refactoring based on the information it has been trained on. However, it cannot directly refactor code. Developers need to implement the suggested changes themselves.
You can use various tools, including integrated development environments (IDEs) such as Visual Studio Code, IntelliJ IDEA, and Eclipse. These IDEs often come with built-in refactoring tools, making the process more efficient.
Developers should follow best practices when integrating code refactoring. Some fundamental guidelines include collaborating with testers, automating the process, refactoring in small steps, troubleshooting and debugging separately, understanding the existing codebase, and setting clear refactoring goals.
One example of refactoring involves enhancing source code structure at a specific location and systematically applying the same improvements to all relevant references across the program. The underlying idea is that making incremental, behavior-preserving changes throughout the codebase has a cumulative positive impact.