What is Bubble Sort and How this algorithm works?
July 13, 2021 Max 4min read
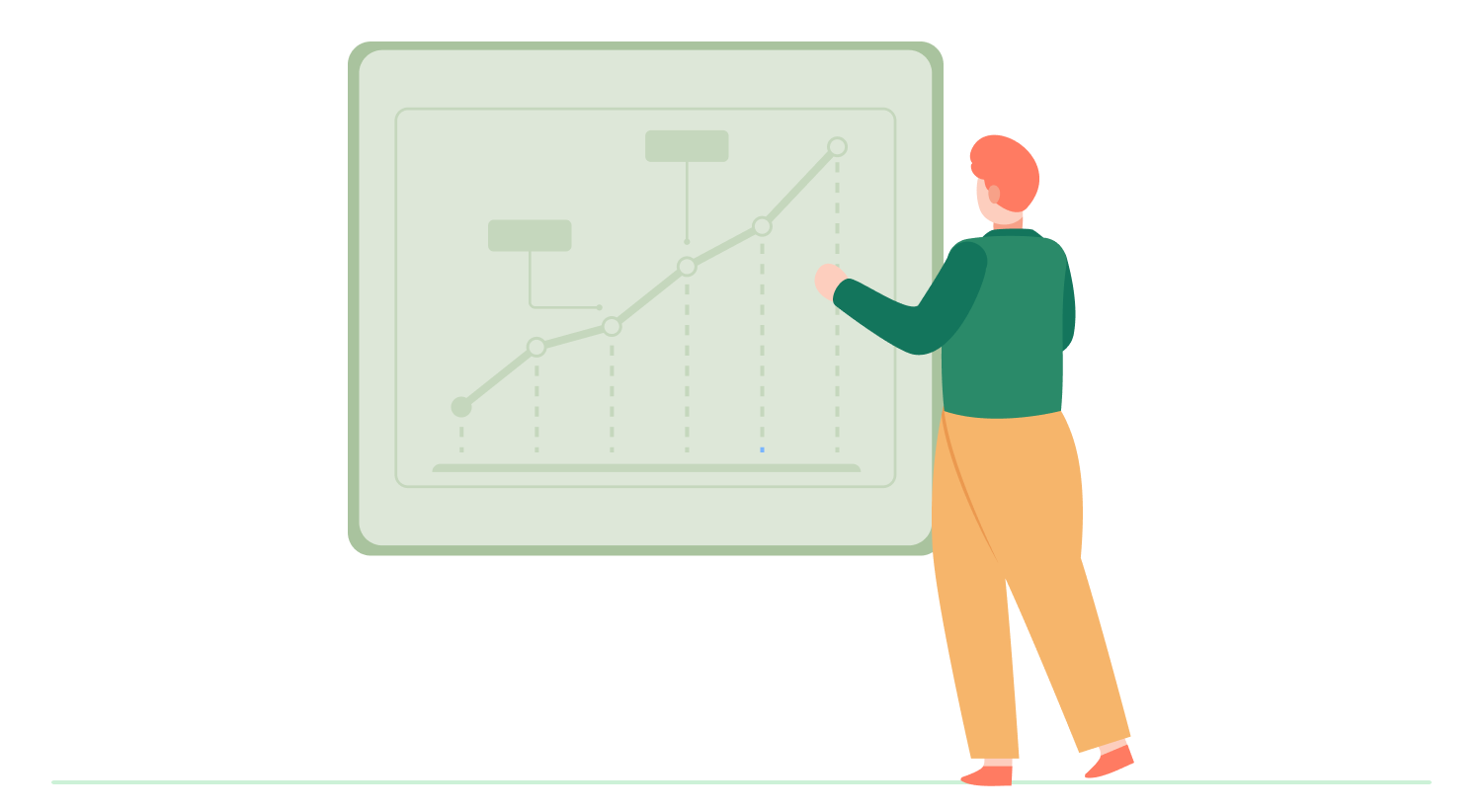
What is Bubble Sort?
Bubble Sort Definition
Bubble sort is a simple algorithm for correctly putting numbers or other elements. The technique examines each group of neighboring elements in the string, from left to right, and switches their locations if they are out of order.
Bubble sort is a fundamental sorting technique used in programming. The bubble sort algorithm moves through the whole dataset multiple times, rearranging them into ascending or descending order.
The algorithm compares an element with the element adjacent to it. Suppose the element is greater than the element next to it.
In that case, they are swapped (if the required overall arrangement is ascending). In this article, we will always consider the arrangement required as ascending.
After every single traversal of the dataset, the greatest element gets automatically placed at the end of the list.
The last element does not appear in the list that gets used to sort after every traversal.
For example if a list is [1,3,4,2,7,5]. The algorithm will first compare 1 and 3. Since 1<3, no swapping occurs. Similarly, for 3 and 4 as well.
When the algorithm arrives at 4 and 2, since 4>2, you swap them, and the list will become [1,3,2,4,7,5].
Further, no swapping occurs at 4 and 7 but 7 and 5. Hence, after a single traversal, the list becomes [1,3,2,4,5,7]. Notice that the largest element 7 automatically arrives at the last place.
For the next traversal, the algorithm will only consider the list as [1,3,2,4,5], neglecting the 7, and then perform bubble sort on the new list.
Suppose you choose to swap elements smaller than the element next to them. In that case, the list will return in descending order.
Benefits and pitfalls of Bubble Sort
One of the most substantial positives about Bubble Sort is its lack of complexity. It is a relatively simple algorithm to code. The only primary task to perform is the comparison of two elements and swapping them if they satisfy the required criteria.
Managers need bubble sort to prioritize work so that the team’s efforts get focused in the right way. It assists the product manager in determining which features are relevant to the client and which strategy will assure success. It also helps understand which product will be the most demanding. That’s with product management software and product roadmap tools.
Bubble Sort, however, is not considered efficient for large pieces of data as it takes a lot of time. In computer programming, bubble sort has a time complexity of O(n log) (n is the number of elements in the dataset). It is not considered very good for efficient coding.
Quicksort vs. Bubble sort
The most basic sorting algorithm is the bubble sort. It entails a series of repetitive sorting of the list. It analyses two adjacent list entries and swaps them if they aren’t right. It goes on until no further exchanges are necessary.
The sorted list’s signal is this. Because it employs comparisons, it is also known as the comparison sort.
Bubble sort is the most basic sorting algorithm strategy. It includes exchanging two neighboring components to position them in the correct order.
Bubble sort is a straightforward algorithm that iterates through a list, comparing neighboring pairings and swapping them out of order. A sinking sort is another name for it.
Quick Sort is the best sorting algorithm that uses the divide-and-conquer strategy. It begins by selecting a ‘pivot’ element to divide the list into two sections.
It then groups elements smaller than the pivot into one sub-list and larger ones into another sub-list while retaining the pivot in its original position.
Quicksort is based on the divide and wins algorithm. A key element is the focal point of division around the provided array.
Quicksort, also known as partition-exchange Sort, is a method of sorting array elements.
Example of how a bubble sort algorithm works?
The following list might get sorted using this algorithm:
13, 12, 14, 11, 15
The algorithm’s first loop would result in:
13, 12, 14, 11, 15 (12<13; thus, the numbers get reversed)
12, 13, 14,11, 15 (13<14. The two numbers are not reversed)
12, 13, 14, 11, 15 (11<14. Thus, the two numbers get swapped)
12, 13, 11, 14, 15 (14<15. No swapping of numbers)
12, 13, 11, 14, 15 (First pass completed)
Due to the swapped value, you must execute the algorithm again. The algorithm’s second loop would begin with the last list and proceed as follows:
12, 13, 11, 14, 15 (12<13. No swapping)
12,11, 13, 11, 14, 15 (11<13 the values are swapped)
12, 11, 13, 14, 15 (13<14. No swapping of values)
12, 11, 13, 14, 15 (14<15. No swapping of values)
12, 11, 13, 14, 15 (Second pass completed)
12, 11, 13, 14, 15 (11<12 the values are reversed)
11, 12, 13, 14, 15 (12<13 , no swapping of values)
11, 12, 13, 14, 15 (13<14. No swapping of numbers)
11, 12, 13, 14, 15 (14<15. No swapping of numbers)
11, 12, 13, 14, 15 (Third pass completed)
Due to the swapped value, you must rerun the algorithm. There will be no swaps this time because the values are in order:
11, 12, 13, 14, 15
Because the algorithm has finished a loop without swapping anything, it recognizes that the list is now ordered and is possible to stop.
Why is the bubble sort inefficient for large arrays?
Because of its inefficiency in sorting large arrays, Bubble Sort has become one of the most commonly discussed algorithms.
After sorting an array, Bubble Sort becomes capable of traversing it. The worst case is an O(N2) run time, which is exceedingly inefficient. It only moves one element at a time in the array. Since items are moved instantly to their final location in the array, they frequently conduct fewer exchanges.
FAQs
Bubble sort is frequently conducted of list entries with higher values than their neighbors’ “bubble.” The largest element, for example, gets bubbled to the rightmost location after the first pass.
It has an average and worst-case running time of O ( n 2 ) O\big(n^2\big) O(n2) and can run in its best-case running time of O ( n ) O(n) O(n) as soon as the input list gets sorted.
Bubble sort is a simple algorithm rearranging elements in ascending or descending order. It’s useful for smaller sets of elements.